Note
This post is about showing the default 404 page with the Next.js Pages Router.
Want to learn how to show and customize the 404 page for the Next.js App Router instead?
Next.js comes with a pretty nice 404 page out of the box. By default it gets shown when Next.js can't find any route for the path you're trying to access.
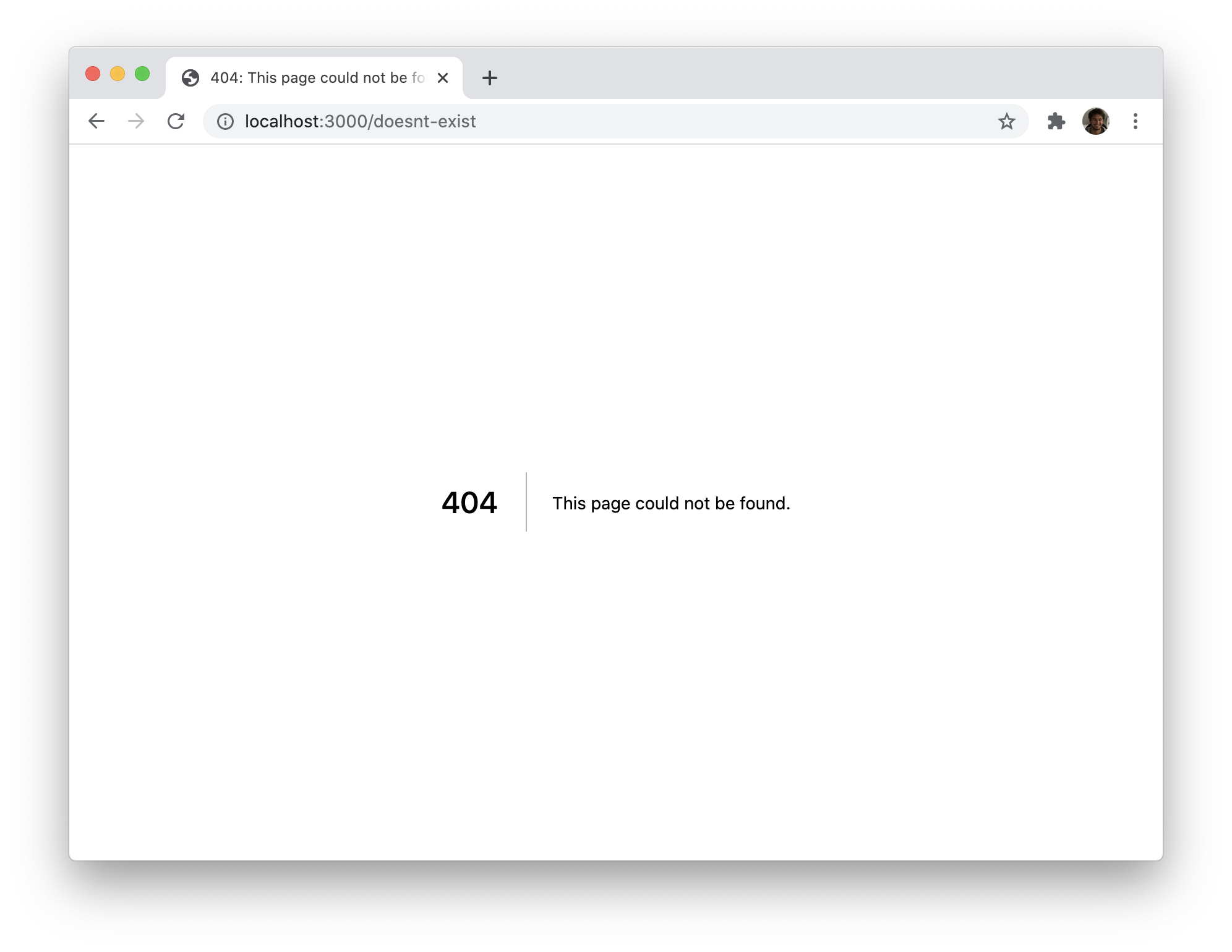
You can also show it programmatically by using the <ErrorPage />
component.
JS
import ErrorPage from 'next/error'async function getInitialProps({ query, res }) {const blogPost = await api.fetchBlogPost(query.slug)if (!blogPost) {if (res) {res.statusCode = 404}return { errorStatus: 404 }}return { blogPost }}function BlogPost({ errorStatus, blogPost }) {if (errorStatus) {return <ErrorPage statusCode={errorStatus} />}return <BlogPost blogPost={blogPost} />}BlogPost.getInitialProps = getInitialPropsexport default BlogPost
If you have a lot of pages where 404s can occur, you might want to move
the ErrorPage rendering to _app.js
.
Optional: Show error page in _app.js
You can also extend _app.js
to magically show the error page if the initial props of a page have an errorStatus
property:
JS
import ErrorPage from 'next/error'import App from 'next/app'async function getInitialProps(appContext) {const { res } = appContext.ctxconst appProps = await App.getInitialProps(appContext)if (appProps.pageProps?.errorStatus && res) {res.statusCode = appProps.pageProps.errorStatus}return {...appProps,}}function MyApp({ Component, pageProps }) {if (pageProps?.errorStatus) {return <ErrorPage statusCode={pageProps.errorStatus} />}return <Component {...pageProps} />}MyApp.getInitialProps = getInitialPropsexport default MyApp